[Spring] xml 시작전, 시작후 동작하는 메소드
- Web / Spring
- 2020. 10. 21.
xml을 불러올 때와 끝났을 때만 불러오는 코드가 있을까?
그 방법에 대해서 xml 방식의 일부를 설명이다.
package com.day02.Ex04;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
import org.springframework.context.support.GenericXmlApplicationContext;
public class MainClass {
public static void main(String [] args)
{
GenericXmlApplicationContext ctx
= new GenericXmlApplicationContext();
ctx.load("classpath:StudentEx04.xml");
ctx.refresh();
ctx.close();
}
}
메인에서 ctx를 실행하고 끝난다면 아래와 같은 실행 문구가 나온다.
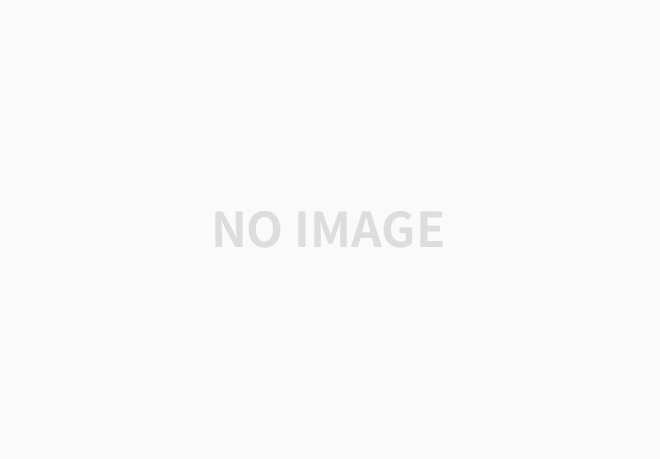
위의 메인 코드를 본다면 아무것도 존재하지 않는다.
그러나 콘솔창에는 이와 같이 문구가 써져 있는 것을 확인 할 수가 있다.
이는 실행도중이 아닌, 실행하기 전과 끝났을 때의 순간에만 실행되는 메소드가 존재해서 이다.
package com.day02.Ex04;
import java.util.*;
import org.springframework.beans.factory.DisposableBean;
import org.springframework.beans.factory.InitializingBean;
public class Student implements InitializingBean, DisposableBean{
private String name;
private int age;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
//빈 소멸과정에서 호출 => ctx.close() 호출
@Override
public void destroy() throws Exception {
// TODO Auto-generated method stub
System.out.println("destroy()");
}
//빈 초기화 과정에서 호출 => ctx.refresh() 호출
@Override
public void afterPropertiesSet() throws Exception {
// TODO Auto-generated method stub
System.out.println("afterPropertiesSet()");
}
}
첫 번째 방식이다.
오버라이드를 사용할 경우에는 implements InitializingBean, DisposableBean 사용한다.
그러면 상속 된 부모클레스가 갖고 있는 afterPropertiesSet 메소드와 destroy 메소드를 사용해야 한다.
그렇다면 오버라이드를 사용 하고 싶지 않을 때에는 어떻게 해야 할까?
package com.day02.Ex04;
import javax.annotation.PostConstruct;
import javax.annotation.PreDestroy;
public class OtherStudent {
private String name;
private int age;
public OtherStudent(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
@PostConstruct //빈 초기화 과정에서 호출 => ctx.refresh() 호출
public void initMethod() {
System.out.println("initMethod()");
}
@PreDestroy //빈 소멸과정에서 호출 => ctx.close() 호출
public void destroyMethod() {
System.out.println("destroyMethod");
}
}
두 번째 방식이다.
오버라이드 대신에 어노테이션을 이용하면 된다.
어노테이션에 @PostConstruct와 @PreDestroy가 있다.
어노테이션이 이를 받아 들여 시작과 끝에 작동이 된다.
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.1.xsd">
<context:annotation-config />
<bean id="student" class="com.day02.Ex04.Student">
<property name="name" value="홍길동" />
<property name="age" value="20" />
</bean>
<bean id="otherStudent" class="com.day02.Ex04.OtherStudent">
<constructor-arg value="홍길자" />
<constructor-arg value="50" />
</bean>
</beans>
대신에 어노테이션을 사용했으므로 중간에 <context:annotation-config /> 를 사용했다는 것을 알려야 한다.
실행은 bean 실행 했을 때 해당 class 내의 실행을 해야 구분이 가능하다.
반응형
'Web > Spring' 카테고리의 다른 글
[Spring] xml 가져오기2(줄임 방식) (0) | 2020.10.22 |
---|---|
[Spring] 외부의 xml 자료를 가져오기 (0) | 2020.10.22 |
[Spring] Xml Java vs XML (0) | 2020.10.21 |
[Spring] xml 활용하기 (0) | 2020.10.21 |
[Spring] xml사용하기1 (0) | 2020.10.20 |